Python is a popular programming language known for its simplicity, readability, and versatility. It is widely used in various fields such as web development, data science, and automation. One of the key features of Python is its ability to handle different data types, including lists and strings. In this article, we will explore the concept of converting lists to strings in Python and the various methods that can be used to achieve this.
What Is a List?
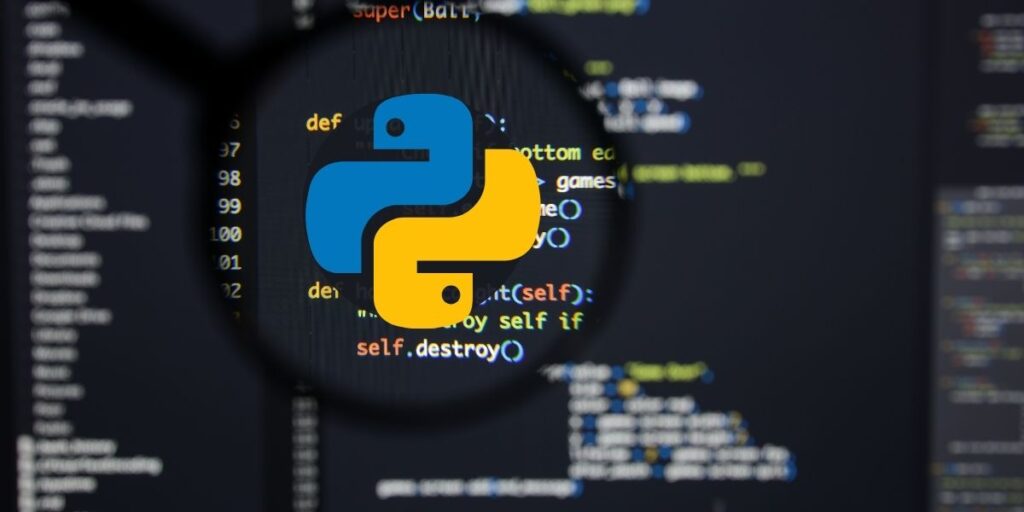
A list is a mutable, ordered sequence that can store items of any data type. It is one of the most commonly used data structures in Python and is used to store related data in a specific order. Lists are denoted by square brackets [ ] and can contain any number of elements separated by commas. Here’s an example of a list containing integers:
numbers = [1, 2, 3, 4, 5]
Lists can also contain elements of different data types, such as strings, floats, and even other lists. They can be modified by adding, removing, or changing elements, making them a versatile tool for data manipulation.
What Is a String?
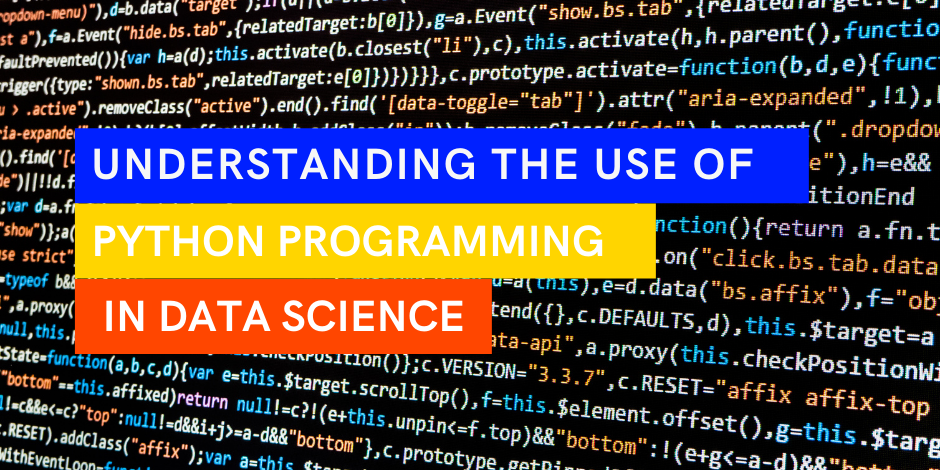
A string is an immutable, ordered sequence of characters. It is used to represent text data and is denoted by single or double quotes. Strings can contain letters, numbers, symbols, and even spaces. Here’s an example of a string:
name = "John Doe"
Strings cannot be modified once they are created, which means that any changes made to a string will result in a new string being created. This property is known as immutability.
Why Convert a Python List to a String?
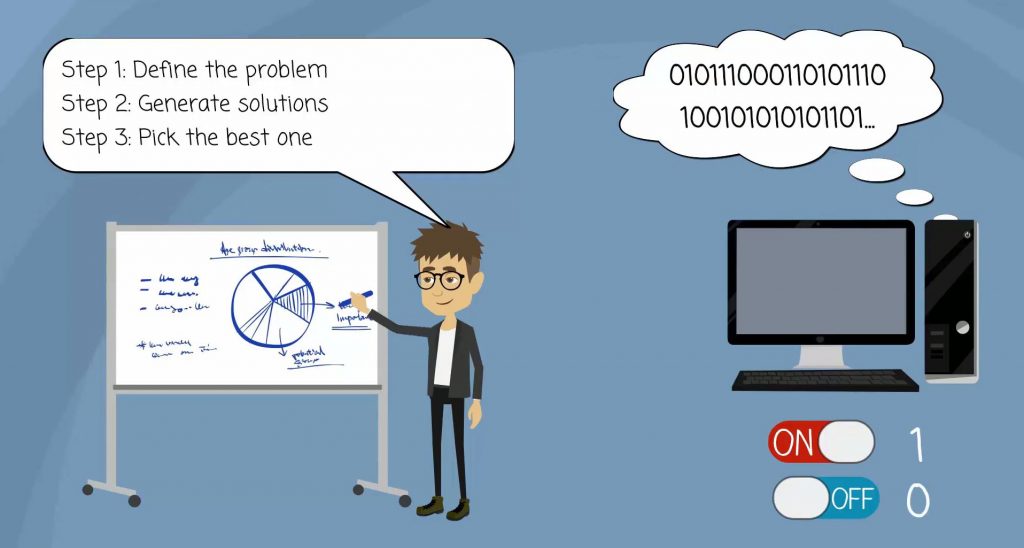
There are several reasons why you might need to convert a Python list to a string. Some of the common use cases include:
- For storage or transmission in a structured format: Many databases and file formats require data to be in a specific format, such as a string. Converting a list to a string allows for easier storage and transmission of data.
- For output formatting: When printing data to the console or writing it to a file, it is often necessary to convert it to a string for proper formatting.
- For compatibility with certain libraries or APIs: Some libraries or APIs may only accept data in the form of a string, making it necessary to convert lists to strings before using them.
Now that we understand the basics of lists and strings, let’s explore the different methods for converting a Python list to a string.
Using the Join Function
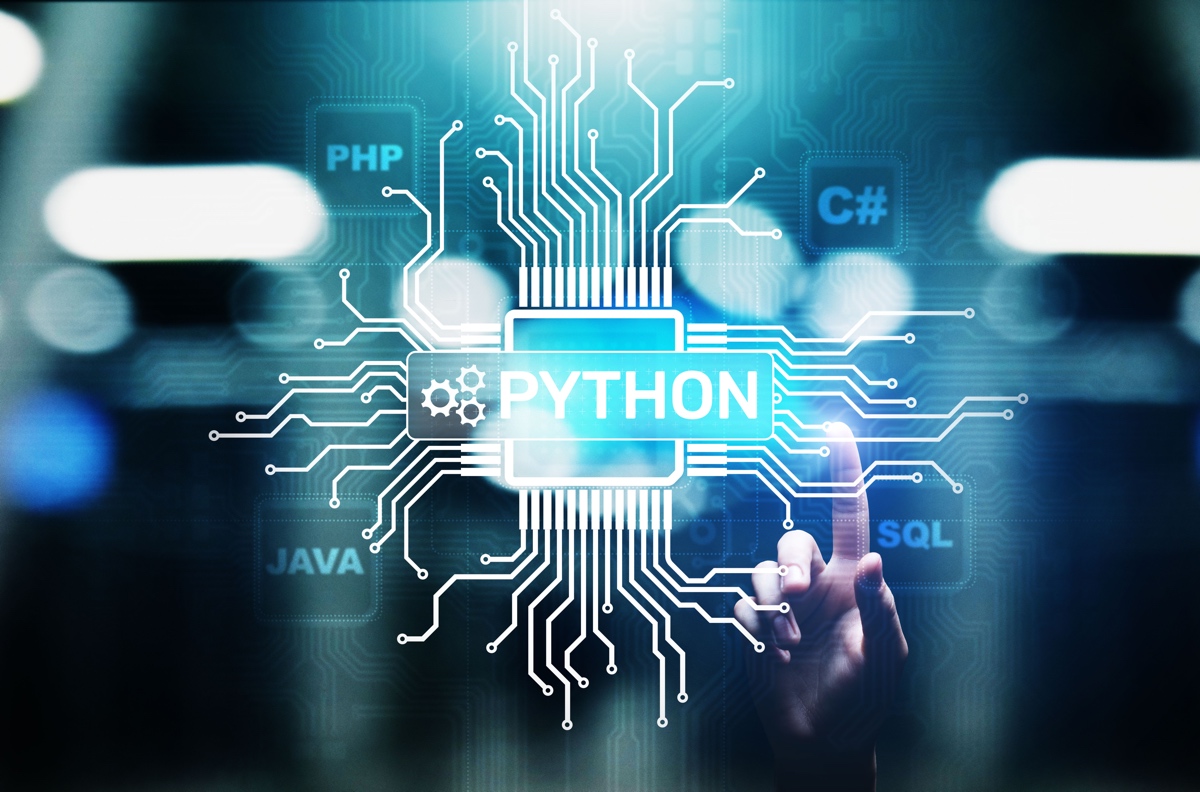
The join() function is a built-in method in Python that can convert a list of strings into a single string. It takes a separator as an argument and joins all the elements of the list together, separated by the specified separator. Here’s the syntax for using the join() function:
string = separator.join(list)
Let’s look at an example to better understand how this works. Say we have a list of names that we want to convert to a string separated by commas. We can use the join() function to achieve this:
names = ["John", "Jane", "Bob", "Alice"]
string = ", ".join(names)
print(string)
# Output: John, Jane, Bob, Alice
In the above example, we used a comma followed by a space as the separator. This resulted in a string where each name was separated by a comma and a space. If we had used a different separator, such as a hyphen, the resulting string would have been different.
It is important to note that the elements in the list must be of type string for the join() function to work correctly. If the elements are of different data types, they must be converted to strings before using the join() function.
Example: Converting a List of Integers to a String
Let’s say we have a list of numbers that we want to convert to a string. Since the elements in this list are integers, we need to convert them to strings before using the join() function. We can achieve this by using the map() function, which we will discuss in more detail later in this article.
numbers = [1, 2, 3, 4, 5]
string = ", ".join(map(str, numbers))
print(string)
# Output: 1, 2, 3, 4, 5
In the above example, we used the map() function to apply the str() function to each element in the list, converting them to strings. The resulting transformed list was then passed to the join() function, resulting in a string where each number was separated by a comma and a space.
Using List Comprehension
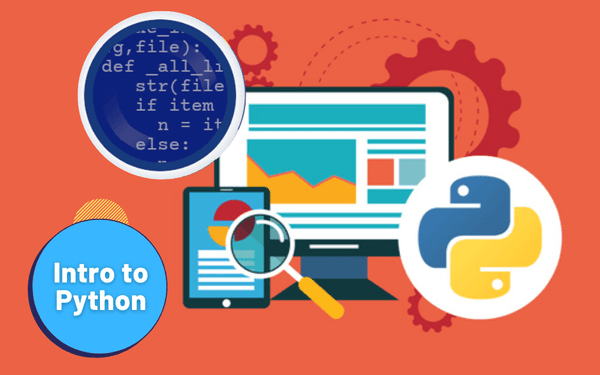
List comprehension is a concise way to create a new list by iterating over the original list and applying a transformation to each element. It follows the syntax of [expression for item in list]
and can also include conditional statements for filtering elements. This makes it a powerful tool for data manipulation in Python.
To convert a list to a string using list comprehension, we can first iterate over the list and convert each element to a string. Then, we can use the join() function to join all the elements together into a single string. Here’s an example:
numbers = [1, 2, 3, 4, 5]
string = ", ".join([str(num) for num in numbers])
print(string)
# Output: 1, 2, 3, 4, 5
In the above example, we used list comprehension to iterate over the numbers list and convert each element to a string. The resulting list was then passed to the join() function, resulting in a string where each number was separated by a comma and a space.
Example: Converting a List of Names to Uppercase
Let’s say we have a list of names that we want to convert to uppercase and join into a single string. We can use list comprehension to achieve this:
names = ["John", "Jane", "Bob", "Alice"]
string = ", ".join([name.upper() for name in names])
print(string)
# Output: JOHN, JANE, BOB, ALICE
In the above example, we used the upper() method to convert each name to uppercase before joining them into a string. This is just one example of how list comprehension can be used for data manipulation.
Using Traversal of a List
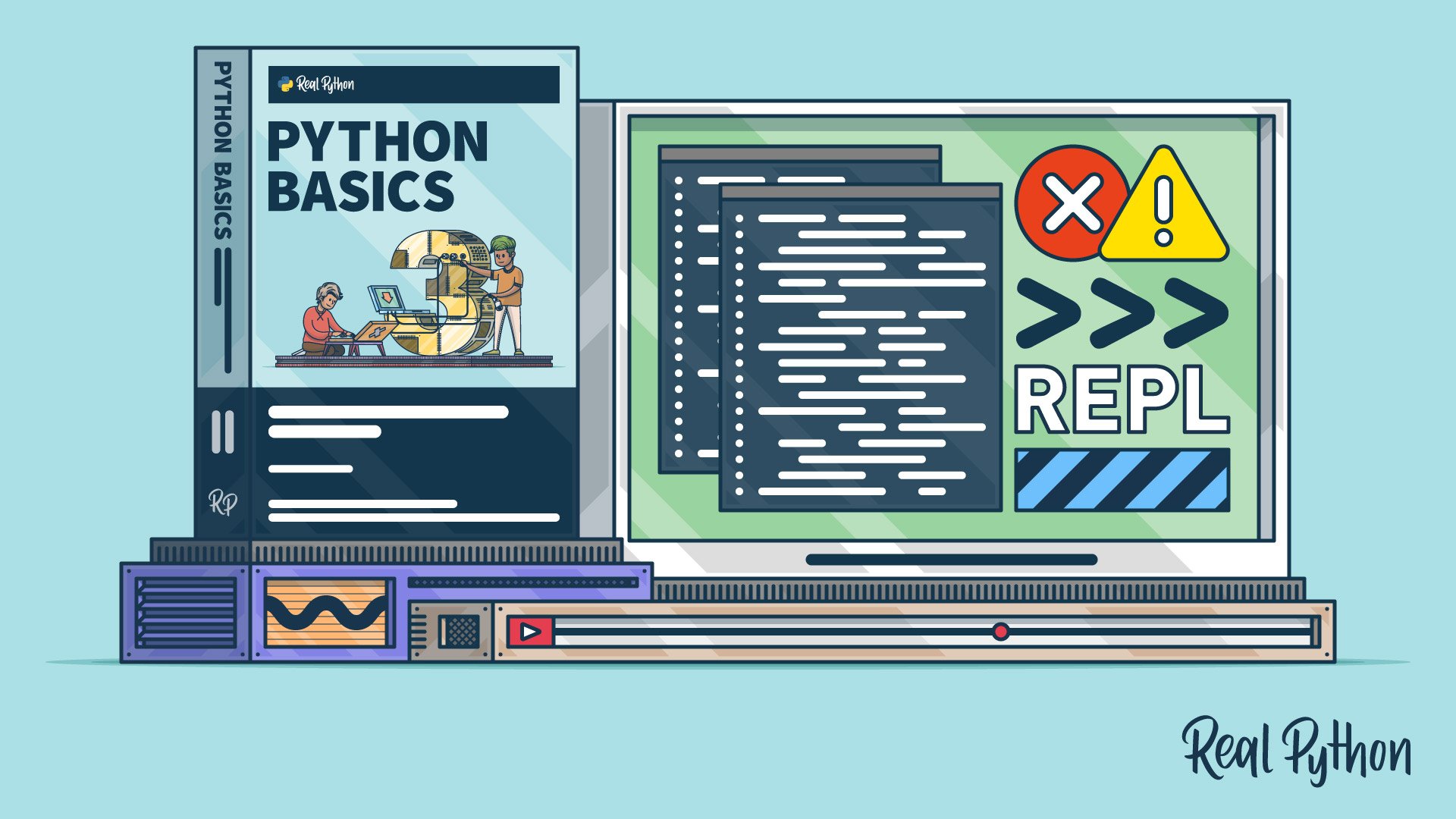
Another way to convert a list to a string is by traversing the list and adding each element to an empty string. This method involves using a loop to iterate over the list and concatenate each element to the string. Here’s an example:
numbers = [1, 2, 3, 4, 5]
string = ""
for num in numbers:
string += str(num) + ", "
print(string[:-2])
# Output: 1, 2, 3, 4, 5
In the above example, we used a for loop to iterate over the numbers list and add each element to the string. The [:-2]
at the end is used to remove the last two characters, which are a comma and a space, from the string. This is necessary because the loop adds a comma and a space after each element, including the last one.
Example: Converting a List of Strings to Title Case
Let’s say we have a list of strings that we want to convert to title case and join into a single string. We can use traversal of the list to achieve this:
names = ["john doe", "jane smith", "bob johnson", "alice brown"]
string = ""
for name in names:
string += name.title() + ", "
print(string[:-2])
# Output: John Doe, Jane Smith, Bob Johnson, Alice Brown
In the above example, we used the title() method to convert each name to title case before adding it to the string. This resulted in a string where each name was separated by a comma and a space.
Using the map() Function
The map() function can be used to apply a transformation to each element in a list. It takes two arguments – a function and an iterable, such as a list. The function is applied to each element in the iterable, and the resulting transformed list is returned. Here’s the syntax for using the map() function:
map(function, iterable)
To convert a list to a string using the map() function, we can first apply the str() function to each element in the list, converting them to strings. Then, we can use the join() function to join all the elements together into a single string. Here’s an example:
numbers = [1, 2, 3, 4, 5]
string = ", ".join(map(str, numbers))
print(string)
# Output: 1, 2, 3, 4, 5
In the above example, we used the map() function to apply the str() function to each element in the numbers list, converting them to strings. The resulting transformed list was then passed to the join() function, resulting in a string where each number was separated by a comma and a space.
Example: Converting a List of Floats to Strings
Let’s say we have a list of floating-point numbers that we want to convert to strings. We can use the map() function to achieve this:
numbers = [1.5, 2.7, 3.9, 4.2, 5.8]
string = ", ".join(map(str, numbers))
print(string)
# Output: 1.5, 2.7, 3.9, 4.2, 5.8
In the above example, we used the map() function to apply the str() function to each element in the numbers list, converting them to strings. The resulting transformed list was then passed to the join() function, resulting in a string where each number was separated by a comma and a space.
Using the str() Function
The str() function can be used to convert each element in a list to a string. It takes an object as an argument and returns its string representation. This makes it useful for converting lists containing elements of different data types to strings. Here’s an example:
numbers = [1, 2, 3, 4, 5]
strings = list(map(str, numbers))
print(strings)
# Output: ['1', '2', '3', '4', '5']
In the above example, we used the map() function to apply the str() function to each element in the numbers list, converting them to strings. The resulting transformed list was then converted back to a list using the list() function.
Example: Converting a List of Booleans to Strings
Let’s say we have a list of boolean values that we want to convert to strings. We can use the str() function to achieve this:
booleans = [True, False, True, False]
strings = list(map(str, booleans))
print(strings)
# Output: ['True', 'False', 'True', 'False']
In the above example, we used the map() function to apply the str() function to each element in the booleans list, converting them to strings. The resulting transformed list was then converted back to a list using the list() function.
Conclusion
Converting lists to strings is a common task in Python and can be achieved using various methods. In this article, we explored five different methods for converting lists to strings – using the join() function, list comprehension, traversal of a list, the map() function, and the str() function. Each method has its advantages and can be used depending on the specific requirements of a program.
We also learned about the basics of lists and strings in Python, including their properties and use cases. Understanding these concepts is essential for effectively working with data in Python and creating efficient programs.
In conclusion, being able to convert lists to strings is an important skill for any Python programmer. With the knowledge gained from this article, you can now confidently handle data manipulation tasks that involve converting lists to strings.