When it comes to programming, data is a crucial element that helps in creating and manipulating information. In simple terms, data can be defined as a collection of facts, figures, or values that are used to represent real-world entities. However, not all data is created equal. In programming, data is classified into two main categories – primitive and non-primitive data types. While primitive data types are the basic building blocks of any programming language, non-primitive data types play a vital role in enhancing the functionality and efficiency of a program.
In this article, we will delve deeper into the world of non-primitive data types, exploring their definition, types, and applications. We will also discuss the differences between primitive and non-primitive data types and how they work together to create robust and dynamic programs. So, let’s get started!
Outline:
- Introduction to Non-Primitive Data Types
- Definition of Non-Primitive Data Types
- Importance of Non-Primitive Data Types in Programming
- Types of Non-Primitive Data Types
- Arrays
- Structures
- Classes
- Applications of Non-Primitive Data Types
- Data Storage and Manipulation
- Object-Oriented Programming
- Dynamic Memory Allocation
- Differences Between Primitive and Non-Primitive Data Types
- Definition
- Size and Memory Allocation
- Mutability
- Working with Non-Primitive Data Types
- Declaring and Initializing Non-Primitive Variables
- Accessing and Modifying Non-Primitive Data
- Common Errors and How to Avoid Them
- Conclusion
Introduction to Non-Primitive Data Types

Non-primitive data types, also known as derived or reference data types, are more complex and sophisticated than primitive data types. They are built upon primitive data types and are used to store and manipulate a collection of data. Unlike primitive data types, which can only hold a single value, non-primitive data types can store multiple values and even other data types within them.
Definition of Non-Primitive Data Types
In simple terms, non-primitive data types can be defined as data structures that are created by combining one or more primitive data types. They are also referred to as derived data types because they are derived from primitive data types. Non-primitive data types are used to represent complex data structures such as lists, arrays, objects, and more.
Importance of Non-Primitive Data Types in Programming
Non-primitive data types play a crucial role in programming as they allow for the creation of more complex and dynamic programs. They provide programmers with the ability to store and manipulate large amounts of data efficiently. Additionally, non-primitive data types enable the implementation of advanced programming concepts such as object-oriented programming, which is widely used in modern software development.
Types of Non-Primitive Data Types
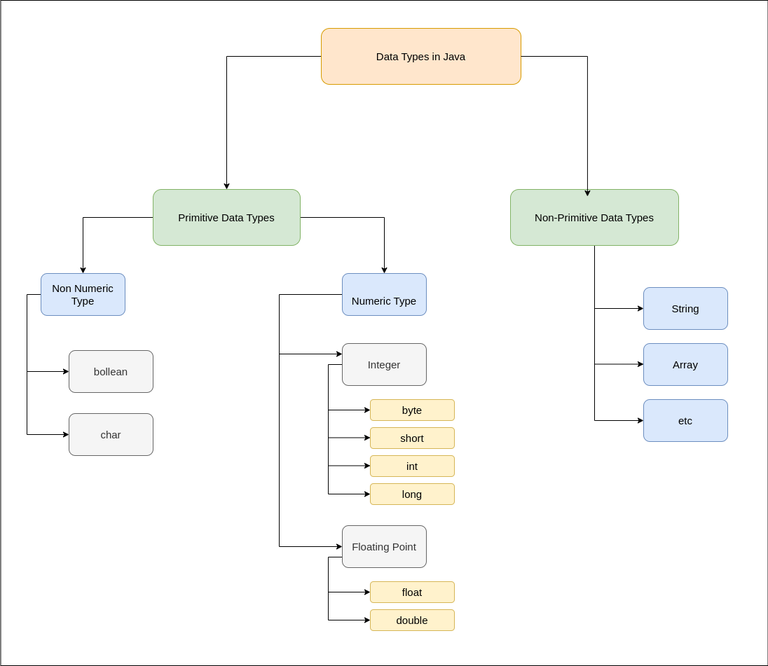
There are several types of non-primitive data types, each with its own unique characteristics and applications. In this section, we will discuss the three most commonly used non-primitive data types – arrays, structures, and classes.
Arrays
Arrays are one of the most fundamental non-primitive data types in programming. They are used to store a collection of elements of the same data type in a contiguous block of memory. Arrays are declared using square brackets []
and can hold a fixed number of elements, depending on the size specified during declaration.
Arrays are incredibly versatile and can be used to store various types of data, including integers, characters, strings, and even other arrays. They are also useful for performing operations on a large set of data, such as sorting, searching, and filtering.
Example:
// Declaring an array of integers
int[] numbers = new int[5];
// Initializing the array with values
numbers[0] = 1;
numbers[1] = 2;
numbers[2] = 3;
numbers[3] = 4;
numbers[4] = 5;
// Accessing and modifying array elements
System.out.println(numbers[2]); // Output: 3
numbers[3] = 10;
System.out.println(numbers[3]); // Output: 10
Structures
Structures, also known as records or tuples, are another type of non-primitive data type that is used to store a collection of related data. Unlike arrays, structures can hold different types of data, making them more flexible and versatile. They are declared using the struct
keyword and can contain multiple fields or variables.
Structures are commonly used in situations where there is a need to group related data together, such as storing information about a person’s name, age, and address. They are also useful for creating custom data types that can be used in various parts of a program.
Example:
// Declaring a structure for a person's information
struct Person {
String name;
int age;
String address;
}
// Initializing a structure variable
Person john = new Person();
john.name = "John";
john.age = 25;
john.address = "123 Main Street";
// Accessing and modifying structure fields
System.out.println(john.name); // Output: John
john.age = 30;
System.out.println(john.age); // Output: 30
Classes
Classes are the most advanced and powerful type of non-primitive data type. They are the building blocks of object-oriented programming and are used to create objects that encapsulate data and behavior. Classes are declared using the class
keyword and can contain attributes (fields) and methods (functions).
Classes are essential in modern programming as they allow for the creation of reusable and modular code. They also enable the implementation of important concepts such as inheritance, polymorphism, and encapsulation, which make programs more efficient and maintainable.
Example:
// Declaring a class for a car
class Car {
String make;
String model;
int year;
// Constructor method
public Car(String make, String model, int year) {
this.make = make;
this.model = model;
this.year = year;
}
// Method to display car information
public void displayInfo() {
System.out.println("Make: " + make);
System.out.println("Model: " + model);
System.out.println("Year: " + year);
}
}
// Creating an instance of the Car class
Car myCar = new Car("Toyota", "Camry", 2020);
// Calling the displayInfo method
myCar.displayInfo();
// Output:
// Make: Toyota
// Model: Camry
// Year: 2020
Applications of Non-Primitive Data Types
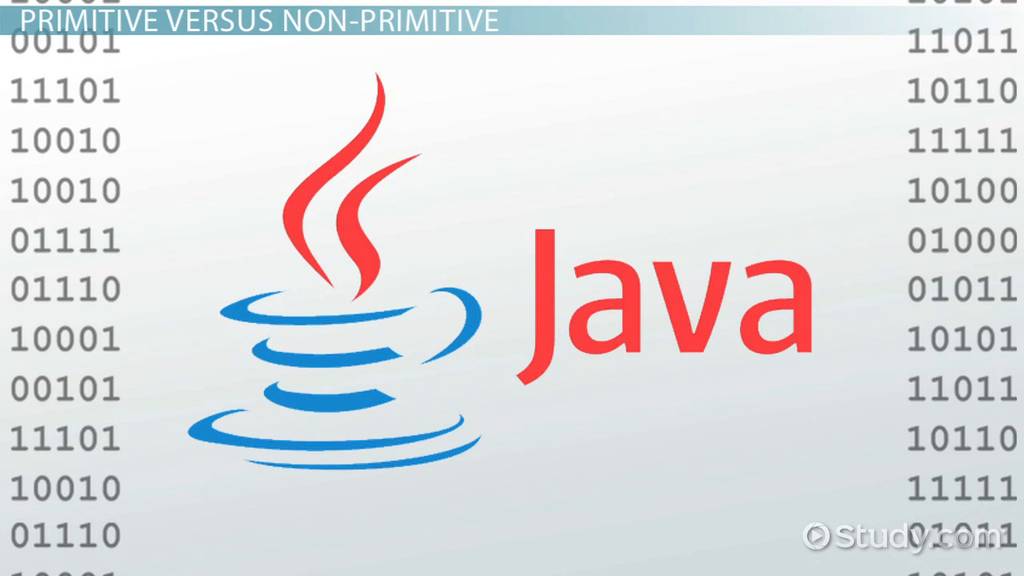
Non-primitive data types have a wide range of applications in programming. In this section, we will discuss some of the most common uses of non-primitive data types.
Data Storage and Manipulation
One of the primary uses of non-primitive data types is to store and manipulate large amounts of data efficiently. Arrays, structures, and classes are all useful in this regard, allowing programmers to organize and access data in a structured manner. This is especially useful when dealing with complex data sets that require frequent manipulation and processing.
Object-Oriented Programming
As mentioned earlier, non-primitive data types play a crucial role in object-oriented programming (OOP). OOP is a programming paradigm that focuses on creating objects that contain both data and behavior. Classes and objects are the building blocks of OOP, and they allow for the creation of modular and reusable code. Non-primitive data types such as structures and classes are essential in implementing OOP concepts such as inheritance, polymorphism, and encapsulation.
Dynamic Memory Allocation
Another significant advantage of non-primitive data types is their ability to allocate memory dynamically. This means that the size of the data structure can be determined at runtime, rather than being fixed during declaration. This is particularly useful when dealing with large or unknown amounts of data, as it allows for more efficient use of memory.
Differences Between Primitive and Non-Primitive Data Types

While both primitive and non-primitive data types serve the purpose of storing and manipulating data, there are some key differences between them. In this section, we will discuss the main differences between these two types of data.
Definition
The most fundamental difference between primitive and non-primitive data types is their definition. Primitive data types are the basic building blocks of a programming language and are defined by the language itself. On the other hand, non-primitive data types are created by combining one or more primitive data types and are not predefined by the language.
Size and Memory Allocation
Another significant difference between primitive and non-primitive data types is their size and memory allocation. Primitive data types have a fixed size and are allocated memory on the stack, which is a limited and fast-access memory space. Non-primitive data types, on the other hand, can vary in size and are allocated memory on the heap, which is a larger but slower-access memory space.
Mutability
Primitive data types are immutable, meaning their values cannot be changed once they are assigned. On the other hand, non-primitive data types are mutable, allowing for the modification of their values. This is because non-primitive data types are stored as references, while primitive data types are stored as values.
Working with Non-Primitive Data Types
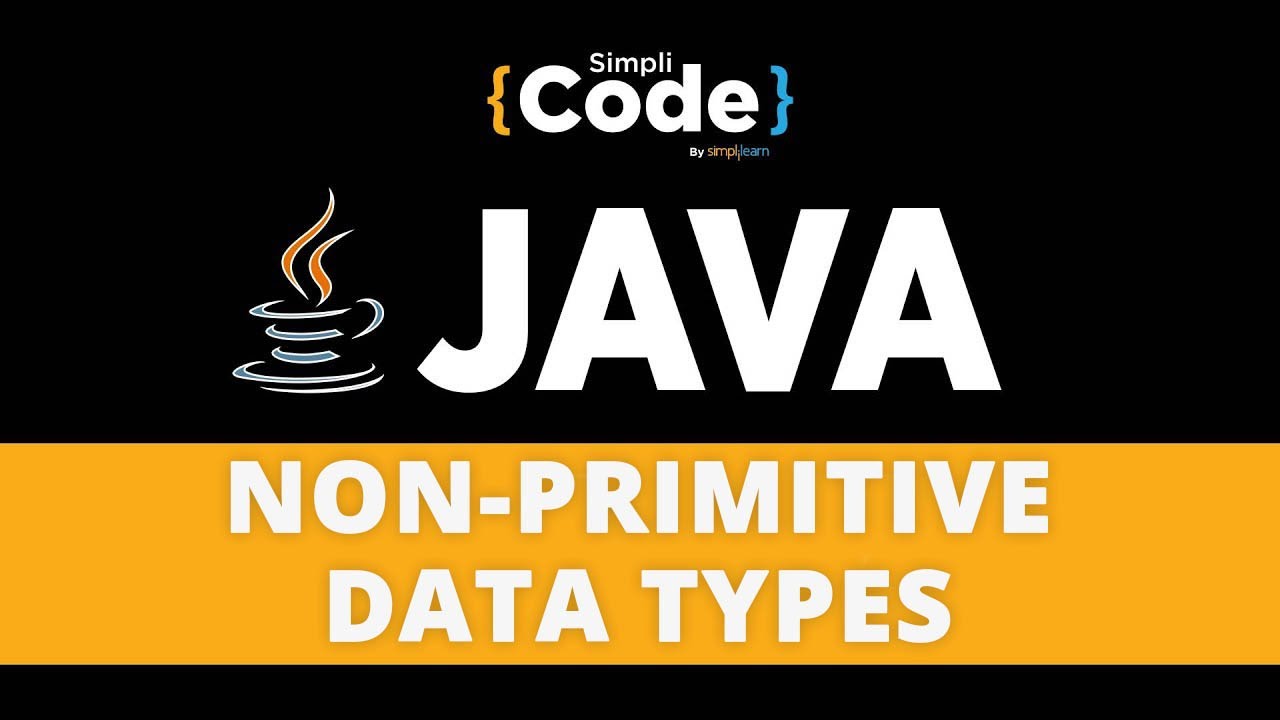
Now that we have a good understanding of non-primitive data types, let’s take a look at how we can work with them in our programs. In this section, we will discuss the steps involved in declaring, initializing, and using non-primitive variables.
Declaring and Initializing Non-Primitive Variables
The first step in working with non-primitive data types is to declare a variable of the desired type. This is done by specifying the data type, followed by the variable name and an optional initial value. For example:
// Declaring an array of integers
int[] numbers;
// Declaring a structure for a person's information
struct Person {
String name;
int age;
String address;
}
// Declaring a class for a car
class Car {
String make;
String model;
int year;
}
Once the variable has been declared, it can be initialized with values using the new
keyword. The syntax for initialization varies depending on the type of non-primitive data type being used. For arrays, the size must be specified during initialization, while structures and classes require the use of a constructor method.
// Initializing an array of integers with 5 elements
numbers = new int[5];
// Initializing a structure variable
Person john = new Person();
john.name = "John";
john.age = 25;
john.address = "123 Main Street";
// Initializing an instance of the Car class
Car myCar = new Car("Toyota", "Camry", 2020);
Accessing and Modifying Non-Primitive Data
Once a non-primitive variable has been declared and initialized, its values can be accessed and modified using dot notation. This means using the variable name, followed by a dot .
and the name of the field or method being accessed. For example:
// Accessing and modifying array elements
System.out.println(numbers[2]); // Output: 3
numbers[3] = 10;
System.out.println(numbers[3]); // Output: 10
// Accessing and modifying structure fields
System.out.println(john.name); // Output: John
john.age = 30;
System.out.println(john.age); // Output: 30
// Calling a method on a class instance
myCar.displayInfo();
// Output:
// Make: Toyota
// Model: Camry
// Year: 2020
Common Errors and How to Avoid Them
Working with non-primitive data types can be tricky, especially for beginners. Here are some common errors that you may encounter when working with non-primitive data types and how to avoid them.
- Null Pointer Exception: This error occurs when trying to access or modify a variable that has not been initialized. To avoid this, make sure to initialize all non-primitive variables before using them.
- Array Index Out of Bounds Exception: This error occurs when trying to access an element in an array at an index that does not exist. To avoid this, make sure to use valid index values when accessing array elements.
- Class Constructor Not Found Error: This error occurs when trying to initialize a class without specifying the required parameters in the constructor method. To avoid this, make sure to provide the necessary arguments when creating an instance of a class.
Conclusion
In conclusion, non-primitive data types are an essential aspect of programming that allows for the creation of more complex and dynamic programs. They provide programmers with the ability to store and manipulate large amounts of data efficiently and enable the implementation of advanced programming concepts such as object-oriented programming. By understanding the different types of non-primitive data types and how they work, you can take your programming skills to the next level and create more robust and efficient programs.